Google Chrome extension to take a screenshot – Requirement
A few days ago at work, I discovered a UI bug in which the button was originally small and then enlarged to its set width. The bug was not reproducible every time, and by the time I snapped the snapshot using the Windows snipping tool, it vanished. So, I discovered a couple of Chrome extensions that allowed me to take screenshots and store them on my local desktop. So this is when I taught myself how to learn and create my own Chrome extensions. You can find the GitHub link to this project at the end of this article.
So what is a Google Chrome Extension?
A Chrome extension is a software application that broadens the capabilities of the Google Chrome web browser by adding extra features and functionalities that is not inbuilt. It is essentially a package of HTML, CSS, JavaScript, and sometimes additional assets that can be easily installed and used within the Chrome browser to add new features, modify existing functionality, or enhance the overall browsing experience.
Through Chrome extensions, developers gain the ability to tailor and enhance different facets of the browser, such as:
- Adding Features: Extensions can add new features and tools to the browser, such as ad blockers, password managers, note-taking tools, or language translators.
- Modifying Web Pages: Extensions can modify the content and appearance of web pages. For example, they can change the appearance of websites, highlight text, or remove elements.
- Automating Tasks: Extensions can automate repetitive tasks, such as filling out forms, capturing screenshots, or managing tabs.
- Customizing User Interface: Extensions can customize the browser’s user interface, such as adding new buttons, changing themes, or adjusting the layout.
Let’s Implement Chrome extension to take a screenshot of the current webpage
We’ll be using HTML, CSS, and JavaScript for this task. Let’s get started:
Set up the project structure:
Create a new folder for your extension and name it something like “screenshot-extension.” Inside this folder, create the following files:
manifest.json
: This file contains metadata about the extension.popup.html
: This file will display the extension’s popup.popup.js
: This file will contain the JavaScript code for taking the screenshot.popup.css
: This file will contain the CSS for styling the popup. Here’s a simplified folder structure for your screenshot Chrome extension:
here’s a simplified folder structure for your screenshot Chrome extension:
screenshot-extension/
│
├── manifest.json
├── popup.html
├── popup.js
├── popup.css
├── icon.png
Coding Time
Update the manifest.json
file.
The manifest.json
file is a central configuration file required for building and packaging Chrome extensions. It provides essential information about the extension, including its name, version, permissions, icons, and other settings. This file serves as a blueprint for the extension, telling the browser how the extension should behave, what resources it requires access to, and how it should be presented to users.
Here’s an overview of some key fields and their purposes within the manifest.json
file:
manifest_version
: Indicates which version of the manifest specification is being used. The current version is typically 2.name
: The name of your extension as it will appear in the Chrome Web Store and the browser.version
: The version number of your extension. This should be updated whenever you make changes to your extension.description
: description of what your extension does. This is displayed in the Chrome Web Store.permissions
: An array of permissions that your extension requires to function. For example, if your extension needs access to tabs, cookies, or storage, you list those permissions here.- action: specifies the behavior of your extension’s icon in the Chrome toolbar. It can define a popup HTML page or a background script to run when the icon is clicked.
content_scripts
: An array of content scripts that your extension can inject into web pages. These scripts can modify or interact with the content of the page.background
: Specifies a background script or a page to run in the background. Background scripts can run even when the extension’s popup is closed.icons
: An object that defines the icons used for your extension at different sizes.permissions
: Specifies the permissions your extension requires. This can include permissions like accessing tabs, cookies, history, and active tabs.content_security_policy
: Defines a content security policy for your extension to mitigate security risks.web_accessible_resources
: Lists resources (files) that are accessible to web pages. This is useful for providing resources to content scripts.
The manifest.json
file is crucial because it outlines how your extension interacts with the browser and web pages, what resources it can access, and what capabilities it has. It essentially acts as the contract between your extension and the Chrome browser’s runtime environment.
Before publishing your extension to the Chrome Web Store or distributing it, make sure your manifest.json
file is accurate and complete, as it plays a significant role in how your extension functions and is perceived by users.
Add the following code to your manifest.json
:
{
"manifest_version": 3,
"name": "Screenshot Extension",
"version": "1.0",
"description": "Takes a screenshot of the current page.",
"permissions": ["activeTab", "tabs"],
"action": {
"default_popup": "popup.html"
},
"icons": {
"48": "icon.png"
},
"content_scripts": [
{
"matches": ["<all_urls>"],
"js": ["popup.js"]
}
]
}
Create the popup.html
file:
Add the following code to popup.html
:
<!DOCTYPE html>
<html>
<head>
<title>Chrome browser Screenshot Extension</title>
<link rel="stylesheet" type="text/css" href="popup.css" />
<script src="popup.js"></script>
</head>
<body>
<button id="screenshotBtn">Take Screenshot</button>
</body>
</html>
Create the popup.css
file:
Add some basic styling to popup.css
:
body {
width: 200px;
height: 100px;
padding: 10px;
}
button {
width: 100%;
height: 30px;
background-color: #007bff;
color: #fff;
border: none;
cursor: pointer;
}
Create the popup.js
file:
Add the following code to popup.js
:
// Function to take a screenshot of the current page.
function takeScreenshot() {
chrome.tabs.captureVisibleTab({ format: "png" }, function (screenshotUrl) {
const link = document.createElement("a");
link.href = screenshotUrl;
link.download = "screenshot.png";
link.click();
});
}
// Attach the event listener to the button.
document.addEventListener("DOMContentLoaded", function () {
const screenshotBtn = document.getElementById("screenshotBtn");
if (screenshotBtn) {
screenshotBtn.addEventListener("click", takeScreenshot);
} else {
console.error("Button not found.");
}
});
Load the extension
- Open Google Chrome and go to
chrome://extensions/
. - Turn on the Developer mode (toggle switch in the top-right corner).
- Click on the “Load unpacked” button.
- Select the folder containing your extension files.
Time to test our Chrome screenshot extension.
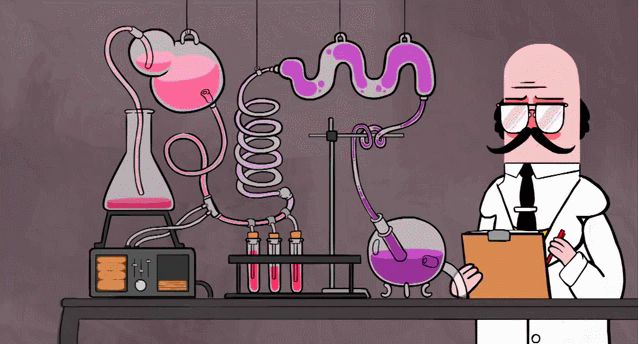
Now, the extension should be installed, and you can see its icon in the Chrome toolbar. Clicking the icon will open the popup with the “Take Screenshot” button. When you click the button, the extension will capture a screenshot of the currently visible tab and save it as “screenshot.png.”
Please note that Chrome extensions have certain limitations when taking screenshots. For example, they cannot capture internal pages, such as the Chrome Web Store or chrome://extensions/. The extension will only work on regular web pages.
Remember to test the extension on various web pages to ensure its functionality. Also, make sure to follow Chrome Web Store policies if you plan to publish it there.
Here is the GitHub link for you to experiment. Happy coding!
Reference
Google Chrome extension getting started
💁 Check out our other articles😃
👉 Generate a free Developer Portfolio website with AI prompts