Introduction
Water droplet animations can add a touch of realism and elegance to your web projects. Whether you’re looking to enhance the visual appeal of your website or create an engaging user experience, mastering the art of water droplet animations is a must. In this comprehensive guide, we’ll show you how to generate mesmerizing water droplet animations using the power of JavaScript, HTML, and CSS. Prepare to captivate your visitors and make a splash online!
You can find the GitHub link at the end of this article
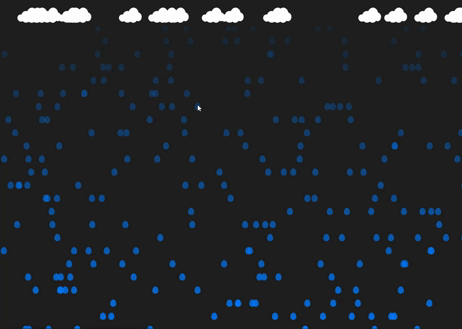
Step 1: Set the Stage with HTML Structure
Before diving into the animation magic, start by setting up the basic HTML structure. Create the foundation for your animation canvas with a few simple elements.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Waterfall Animation</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<div class="clouds">
<!-- Clouds will be added here dynamically using JavaScript -->
</div>
<div class="waterfall">
<div class="water">
<!-- Droplets will be dynamically added here using JavaScript -->
</div>
</div>
<audio id="cloudsAudio" loop>
<source src="clouds.mp3" type="audio/mpeg" />
Your browser does not support the audio element.
</audio>
<script src="script.js"></script>
</body>
</html>
Step 2: Styling the Clouds and Sky
Learn how to create dreamy clouds that provide a picturesque backdrop for your water droplet animation. Harness the power of CSS to achieve cloud movement and a realistic sky-like feel.
Creating clouds
// Create and animate clouds
function createClouds() {
// This line selects the element with the class clouds, which is the container where the cloud elements will be placed.
const cloudsContainer = document.querySelector(".clouds");
for (let i = 1; i <= numClouds; i++) {
const cloud = document.createElement("div");
cloud.classList.add("cloud", `cloud${i}`); // Adds the class cloud to the newly created div.
cloud.style.left = `${getRandomPosition()}px`; // Sets the horizontal position of the cloud using the left CSS property.
cloudsContainer.appendChild(cloud); // Appends the newly created cloud div to the cloudsContainer
animateCloud(cloud, i); // Calls the animateCloud function to animate the cloud's movement.
}
}
// Animate a cloud's movement
function animateCloud(cloud, index) {
const animationDuration = 15 + index * 2; // Adjust the duration as needed
const keyframes = [
{ transform: `translateX(-50%)` },
{ transform: `translateX(50%)` },
{ transform: `translateX(-50%)` },
];
// Apply continuous animation using the Web Animations API
cloud.animate(keyframes, {
duration: animationDuration * 1000, // Convert seconds to milliseconds
iterations: Infinity, // Repeat indefinitely
});
}
Step 3: Craft the Droplets: A Symphony of Animation and Timing
Discover the secret behind those realistic droplets falling from the sky. Dive into JavaScript to dynamically generate and animate water droplets with varying speeds and delays.
Creating droplets
The createDroplets
function is responsible for generating and appending water droplet elements to the animation container. Let’s break down what each step of this function is doing:
// Create droplets with animation delays
function createDroplets() {
// This line selects the element with the class water, which is the container where the droplets will be placed.
const waterContainer = document.querySelector(".water");
// This initiates a loop that will run numDroplets times, where numDroplets is a variable representing the number of droplets you want to create.
for (let i = 0; i < numDroplets; i++) {
//Adds the class droplet to the newly created div. This class will apply the styling to make the element look like a water droplet.
const droplet = document.createElement("div");
droplet.classList.add("droplet");
droplet.style.left = `${getRandomPosition()}px`;
droplet.style.animationDelay = `${i * 0.1}s`; // Add delay for animation variation
waterContainer.appendChild(droplet);
}
}
Step 4: Enchant with Sound: Background Audio for Full Immersion
Immerse your audience even further by incorporating soothing audio. Learn how to embed audio elements into your animation for a multisensory experience that will leave a lasting impression.
// When the DOM is fully loaded
document.addEventListener("DOMContentLoaded", function () {
// Create and animate clouds
createClouds();
// Create droplets with animation delays
createDroplets();
const cloudsAudio = document.getElementById("cloudsAudio");
let isPlaying = false;
// Toggle audio playback on click
document.addEventListener("click", function () {
if (isPlaying) {
cloudsAudio.pause();
isPlaying = false;
} else {
cloudsAudio.play();
isPlaying = true;
}
});
});
<audio id="cloudsAudio" loop>
<source src="clouds.mp3" type="audio/mpeg" />
Your browser does not support the audio element.
</audio>
Step 5: Bringing it All Together: Polishing Your Water Droplet Masterpiece
Fine-tune your animation, adjust timings, and tweak styling to perfection. Achieve a seamless integration of all elements to create a truly breathtaking water droplet animation that wows.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>Waterfall Animation</title>
<link rel="stylesheet" href="styles.css" />
</head>
<body>
<div class="clouds">
<!-- Clouds will be added here dynamically using JavaScript -->
</div>
<div class="waterfall">
<div class="water">
<!-- Droplets will be dynamically added here using JavaScript -->
</div>
</div>
<audio id="cloudsAudio" loop>
<source src="clouds.mp3" type="audio/mpeg" />
Your browser does not support the audio element.
</audio>
<script src="script.js"></script>
</body>
</html>
// script.js
// Number of droplets and clouds
const numDroplets = 300;
const numClouds = 20;
// When the DOM is fully loaded
document.addEventListener("DOMContentLoaded", function () {
// Create and animate clouds
createClouds();
// Create droplets with animation delays
createDroplets();
const cloudsAudio = document.getElementById("cloudsAudio");
let isPlaying = false;
// Toggle audio playback on click
document.addEventListener("click", function () {
if (isPlaying) {
cloudsAudio.pause();
isPlaying = false;
} else {
cloudsAudio.play();
isPlaying = true;
}
});
});
// Create and animate clouds
function createClouds() {
const cloudsContainer = document.querySelector(".clouds");
for (let i = 1; i <= numClouds; i++) {
const cloud = document.createElement("div");
cloud.classList.add("cloud", `cloud${i}`);
cloud.style.left = `${getRandomPosition()}px`;
cloudsContainer.appendChild(cloud);
animateCloud(cloud, i);
}
}
// Create droplets with animation delays
function createDroplets() {
const waterContainer = document.querySelector(".water");
for (let i = 0; i < numDroplets; i++) {
const droplet = document.createElement("div");
droplet.classList.add("droplet");
droplet.style.left = `${getRandomPosition()}px`;
droplet.style.animationDelay = `${i * 0.1}s`; // Add delay for animation variation
waterContainer.appendChild(droplet);
}
}
// Animate a cloud's movement
function animateCloud(cloud, index) {
const animationDuration = 15 + index * 2; // Adjust the duration as needed
const keyframes = [
{ transform: `translateX(-50%)` },
{ transform: `translateX(50%)` },
{ transform: `translateX(-50%)` },
];
// Apply continuous animation using the Web Animations API
cloud.animate(keyframes, {
duration: animationDuration * 1000, // Convert seconds to milliseconds
iterations: Infinity, // Repeat indefinitely
});
}
// Get a random horizontal position within the window width
function getRandomPosition() {
return Math.random() * window.innerWidth;
}
/* styles.css */
body {
margin: 0;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
background-color: #1f1f1f;
}
.clouds {
position: absolute;
top: 20px;
left: 0;
width: 100%;
height: 200px;
overflow: hidden;
}
.cloud {
width: 100px;
height: 40px;
background-image: url("cloud.svg"); /* Replace with your SVG file path */
background-size: contain;
background-repeat: no-repeat;
position: absolute;
}
/* Add more cloud animations here */
@keyframes cloudAnimation1 {
0%,
100% {
transform: translateX(-20%);
}
50% {
transform: translateX(20%);
}
}
@keyframes cloudAnimation2 {
0%,
100% {
transform: translateX(-20%);
}
50% {
transform: translateX(20%);
}
}
/* Add more cloud animation keyframes here */
.waterfall {
width: 100vw;
height: 85vh;
overflow: hidden;
position: relative;
}
.water {
display: flex;
flex-wrap: nowrap;
width: max-content;
position: absolute;
top: 0;
}
.droplet {
width: 16px;
height: 24px;
border-radius: 50% 50% 50% 50% / 60% 60% 40% 40%;
background-color: #007bff;
opacity: 0;
animation: dropAnimation 3s linear infinite;
position: absolute;
transform: translateY(-100px) scaleY(0.8);
}
@keyframes dropAnimation {
0% {
transform: translateY(-100px) scaleY(0.8);
opacity: 0;
}
100% {
transform: translateY(100vh) scaleY(0.8);
opacity: 1;
}
}
.splash-container {
position: absolute;
bottom: 0;
width: 100%;
height: 100%;
overflow: hidden;
}
.splash {
position: absolute;
bottom: 0;
width: 20px;
height: 20px;
border-radius: 50%;
background-color: #007bff;
transform-origin: bottom;
animation: splashAnimation 1s ease-out;
}
@keyframes splashAnimation {
0% {
transform: scale(0);
opacity: 1;
}
100% {
transform: scale(1);
opacity: 0;
}
}
// cloud.svg
<?xml version="1.0" standalone="no"?>
<!DOCTYPE svg PUBLIC "-//W3C//DTD SVG 20010904//EN"
"http://www.w3.org/TR/2001/REC-SVG-20010904/DTD/svg10.dtd">
<svg version="1.0" xmlns="http://www.w3.org/2000/svg"
width="1280.000000pt" height="855.000000pt" viewBox="0 0 1280.000000 855.000000"
preserveAspectRatio="xMidYMid meet">
<metadata>
Created by potrace 1.15, written by Peter Selinger 2001-2017
</metadata>
<g transform="translate(0.000000,855.000000) scale(0.100000,-0.100000)"
fill="#ffffff" stroke="none">
<path d="M8130 8543 c-19 -1 -84 -8 -145 -13 -873 -83 -1730 -711 -2261 -1654
-42 -76 -106 -201 -141 -279 -35 -78 -69 -141 -76 -141 -6 1 -87 32 -179 68
-299 118 -473 148 -736 128 -427 -33 -806 -163 -1167 -400 -320 -209 -616
-513 -820 -842 -94 -152 -201 -383 -260 -564 -30 -91 -55 -172 -55 -181 0 -19
-8 -19 -126 6 -346 72 -827 -7 -1181 -196 -282 -150 -528 -380 -691 -647 -311
-508 -377 -1188 -176 -1788 194 -577 623 -1042 1161 -1259 256 -104 422 -135
703 -135 185 0 217 2 333 27 155 33 359 99 518 168 l119 51 87 -80 c302 -274
627 -471 981 -593 237 -81 440 -121 711 -139 589 -40 1171 123 1739 487 l83
53 97 -86 c303 -268 683 -442 1117 -511 153 -24 492 -24 625 0 471 86 872 311
1237 690 105 110 252 294 317 398 l29 46 171 -82 c307 -148 455 -194 648 -202
576 -24 1148 277 1523 802 448 627 600 1547 394 2380 -211 854 -779 1491
-1494 1675 -64 17 -127 30 -140 30 -13 0 -26 4 -29 9 -4 5 -15 60 -26 123
-221 1269 -1084 2289 -2175 2573 -257 67 -527 96 -715 78z"/>
</g>
</svg>
👉Github link – Waterfall animation👈
💁 Check out our other articles😃
👉 Generate a free Developer Portfolio website with AI prompts
👉 Creating a Toggle Switcher with Happy and Sad Faces using HTML, CSS, and JavaScript
Step 6: Ready for Prime Time: Deploying Your Animation
Unlock the final step of your journey – deploying your water droplet animation masterpiece onto the web. From hosting to sharing, we cover the best practices for showcasing your creation.
Conclusion: Making a Splash Online with Water Droplet Animations
With the power of JavaScript, HTML, and CSS at your fingertips, you now have the tools to craft captivating water droplet animations that engage and delight visitors. From realistic droplets to animated splashes, this step-by-step guide has equipped you to create an impressive animation that will make waves on the web.
Unlock your creative potential and seize the opportunity to make your website truly stand out with this enchanting and visually stunning animation technique. Start your journey today and watch your web projects come to life with the beauty of water droplet animations. Dive in and let your creativity flow!
Now’s the time to turn your vision into reality. Let’s get started and create an unforgettable water droplet animation that leaves a lasting impression!