Docker Compose has revolutionized how developers manage multi-container applications. Whether you’re building a complex microservices architecture or setting up a simple development environment, Docker Compose simplifies the process of defining and running multi-container Docker applications. This comprehensive guide will walk you through everything you need to know about Docker Compose, from basic concepts to practical implementation.
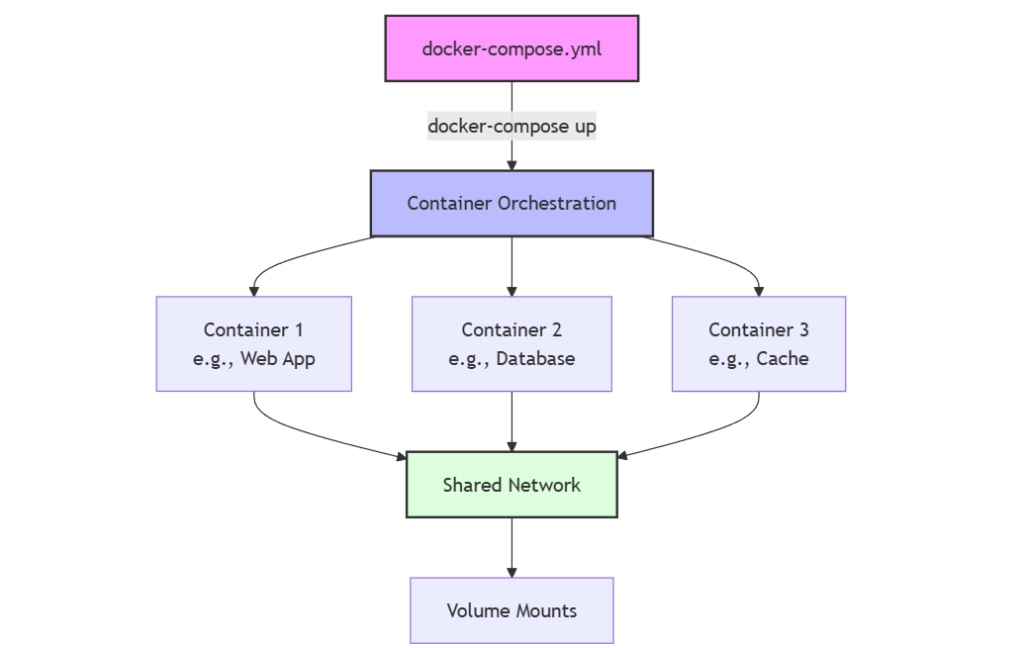
Understanding Docker Compose
Docker Compose is a tool for defining and running multi-container Docker applications. It uses YAML files to configure application services and performs the creation and start-up of all the containers with a single command. Born out of the Fig project in 2014, Docker Compose has become an essential tool in the Docker ecosystem. For more detailed information about its evolution and capabilities, you can refer to the official Docker Compose documentation.
Key concepts of Docker Compose include:
- Services: The different containers that make up your application (Learn more about Docker services)
- Networks: How containers communicate with each other (See Docker networking)
- Volumes: Where persistent data is stored (Explore Docker volumes)
- Environment Variables: Configuration that can change between deployments (Check environment variables in Compose)
Setting Up Docker Compose
Installing Docker
Before installing Docker Compose, you’ll need to have Docker installed on your system. The official installation guide provides detailed instructions for all major platforms. Here’s how to install Docker on different operating systems:
For Ubuntu:
# Update package index
sudo apt-get update
# Install required packages
sudo apt-get install apt-transport-https ca-certificates curl software-properties-common
# Add Docker's official GPG key
curl -fsSL https://download.docker.com/linux/ubuntu/gpg | sudo apt-key add -
# Add Docker repository
sudo add-apt-repository "deb [arch=amd64] https://download.docker.com/linux/ubuntu $(lsb_release -cs) stable"
# Install Docker
sudo apt-get update
sudo apt-get install docker-ce
For Docker Compose installation, follow the official Compose installation guide:
sudo curl -L "https://github.com/docker/compose/releases/latest/download/docker-compose-$(uname -s)-$(uname -m)" -o /usr/local/bin/docker-compose
sudo chmod +x /usr/local/bin/docker-compose
For macOS and Windows, you can simply download Docker Desktop, which includes both Docker Engine and Docker Compose.
Building Your First Example with Docker Compose
Let’s create a simple web application with a Python Flask frontend and a Redis backend to demonstrate Docker Compose in action. You can find more example applications in the Docker samples repository.
Project structure:
./my-flask-app/
├── docker-compose.yml
├── app/
│ ├── Dockerfile
│ ├── requirements.txt
│ └── app.py
First, create the Flask application (app/app.py
):
from flask import Flask
from redis import Redis
app = Flask(__name__)
redis = Redis(host='redis', port=6379)
@app.route('/')
def hello():
redis.incr('hits')
return f'Hello! This page has been viewed {redis.get("hits").decode("utf-8")} times.'
if __name__ == "__main__":
app.run(host="0.0.0.0", debug=True)
Create the requirements file (app/requirements.txt
):
flask
redis
Create the Dockerfile (app/Dockerfile
) following Dockerfile best practices:
FROM python:3.9-slim
WORKDIR /app
COPY requirements.txt .
RUN pip install -r requirements.txt
COPY . .
CMD ["python", "app.py"]
Finally, create the Docker Compose file (docker-compose.yml
) using the Compose file reference:
version: '3'
services:
web:
build: ./app
ports:
- "5000:5000"
volumes:
- ./app:/app
depends_on:
- redis
redis:
image: redis:alpine
volumes:
- redis_data:/data
volumes:
redis_data:
Running the Application
To start the application, run:
docker-compose up
For more detailed command-line options, refer to the Docker Compose CLI reference.
Best Practices and Tips for Using Docker Compose
- Version Control
- Always specify the version of Docker Compose file format (See Compose file versions)
- Use version control for your Docker Compose files
- Document any special requirements or configurations
- Security
- Never commit sensitive data in Docker Compose files
- Use environment variables for sensitive information (Learn about secrets management)
- Implement proper access controls for volumes
- Performance
- Use build cache effectively (See build performance best practices)
- Minimize the number of layers in your Dockerfile
- Use appropriate volume mounts for development
- Networking
- Use custom networks for better isolation (Read about Docker networking)
- Name your networks meaningfully
- Control external access through ports carefully
- Development vs Production
- Maintain separate compose files for development and production (See extending Compose files)
- Use docker-compose.override.yml for development-specific settings
- Consider using production-grade orchestration tools like Kubernetes for production
Conclusion
Docker Compose is an invaluable tool for developers working with containerized applications. It simplifies the process of managing multiple containers and their interactions, making it easier to develop, test, and deploy complex applications. By following the best practices and examples outlined in this guide, you’ll be well-equipped to start using Docker Compose in your own projects.
For further learning, consider exploring:
- Docker Compose CLI environment variables
- Docker Compose networking in depth
- Docker Compose production deployment
- Docker security best practices
Remember that Docker Compose is just one piece of the container orchestration puzzle, but it’s an excellent starting point for developers looking to streamline their development workflow and manage multi-container applications effectively.