Introduction – Fix Spelling Mistakes in Python
In this article, we’ll learn how to auto-correct miss-spelled words using pyspellchecker library. Discover how this library can help you effortlessly identify and rectify misspelled words, boosting the quality of your text and elevating your online credibility.
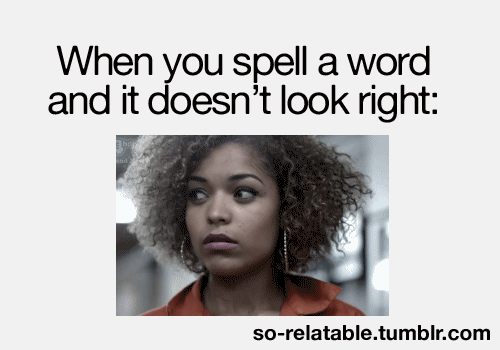
Understanding the Spell Checking Landscape
The art of spell checking involves identifying words that are absent from a predetermined dictionary. Traditional spell checkers rely on static lexicons to verify word correctness. However, the pyspellchecker
library takes a more dynamic approach by harnessing the power of the Levenshtein Distance algorithm, also known as the Edit Distance algorithm, which measures the similarity between two strings by calculating the minimum number of single-character edits required to transform one string into the other. In simpler terms, it’s like finding the shortest path to transform “kitten” into “sitting” โ changing just a few letters at a time.
Fix Spelling Mistakes in Python code implementation
Let’s dive into an example to illustrate the effectiveness of the pyspellchecker
library. Imagine you have a text riddled with potential misspelled words:
pip install pyspellchecker
from spellchecker import SpellChecker
def correct_spelling(text):
# Create a SpellChecker instance
spell = SpellChecker()
# Split the input text into words
words = text.split()
corrected_words = []
for word in words:
# Check if the word is misspelled
if spell.unknown([word]):
# Get the corrected version of the word
corrected_word = spell.correction(word)
corrected_words.append(corrected_word)
else:
corrected_words.append(word)
# Join the corrected words back into a sentence
corrected_text = " ".join(corrected_words)
return corrected_text
# Input text with potentially misspelled words
input_text = "Ths is a proofessional text with some misspeled wrds."
# Correct the spelling in the input text
corrected_text = correct_spelling(input_text)
print("Original text:", input_text)
print("Corrected text:", corrected_text)
Output
Original text: Ths is a proofessional text with some misspeled wrds.
Corrected text: the is a professional text with some misspelled words
It also supports other languages like English – โenโ, Spanish – โesโ, French – โfrโ,Portuguese – โptโ, German – โdeโ, Russian – โruโ, Arabic – โarโ, Basque – โeuโ, Latvian – โlvโ
To set a language: Let’s try to correct spelling in Python for Spanish text
spell = SpellChecker(language='es') // use the short language code
from spellchecker import SpellChecker
# Create a SpellChecker instance
spell = SpellChecker(language='es') # 'es' for Spanish
# Input text in Spanish with potential misspellings
input_text = "Este es un ejemple de texto con algunas palbras mal escrtas."
# Split the input text into words
words = input_text.split()
corrected_words = []
for word in words:
# Check if the word is misspelled
if spell.unknown([word]):
# Get the corrected version of the word
corrected_word = spell.correction(word)
corrected_words.append(corrected_word)
else:
corrected_words.append(word)
# Join the corrected words back into a sentence
corrected_text = ' '.join(corrected_words)
print("Original text:", input_text)
print("Corrected text:", corrected_text)
Original text: Este es un ejemple de texto con algunas palbras mal escrtas.
Corrected text: Este es un ejemplo de texto con algunas palabras mal escritas
๐ Check out our other articles๐
๐ Generate a free Developer Portfolio website with AI prompts
๐ Creating a Toggle Switcher with Happy and Sad Faces using HTML, CSS, and JavaScript
Other libraries that support spell checking in Python and correction
- PyEnchant:
- Provides access to the Enchant spellchecking system, supporting various backends.
- Offers multilingual spell checking capabilities.
- Installation:
pip install pyenchant
.
- NLTK (Natural Language Toolkit):
- A comprehensive NLP library that includes tools for spell checking.
- Offers a wide range of natural language processing functionalities.
- Installation:
pip install nltk
.
- TextBlob:
- Built on top of NLTK, offers a simplified API for NLP tasks including spell checking.
- Great for users seeking ease of use and quick integration.
- Installation:
pip install textblob
.
- LanguageTool-Py:
- Utilizes the LanguageTool proofreading tool for grammar, style, and spell checking.
- Provides a Python interface to LanguageTool’s API.
- Installation:
pip install language-tool-python
.
- aspell-python:
- Integrates the Aspell command-line spell checker with your Python code.
- Suitable for those familiar with the Aspell utility.
- Installation:
pip install aspell-python-py3
.